티스토리 뷰
1. Wrapper 개요
1-1. Wrapper 클래스란?
💡 기본 타입의 데이터를 인스턴스화 해야 하는 경우에 사용.
예를 들어 특정 메소드가 매개변수로 객체 타입만 요구하게 되면 기본 타입의 데이터를 먼저 인스턴스로 변환 후 넘겨줘야한다.
이 때 8가지에 해당하는 기본 타입의 데이터를 인스턴스화 할 수 있도록 하는 클래스를 래퍼클래스(Wrapper class)라고 한다.
(주방에서 사용하는 랩(Wrap)과 같은 의미로 감싼다는 의미)
1-2. 래퍼 클래스 종류
기본 타입 | 래퍼 클래스 |
byte | Byte |
short | Short |
int | Integer |
long | Long |
float | Float |
double | Double |
char | Character |
boolean | Boolean |
📌 래퍼 클래스 장점
1. 기능, 속성 가질 수 있다.
2. Object의 자손이다!
a. equals, hashCode 받을 수 있다.
b. 동등 비교 가능 → constant pool 사용
3. 다형성 적용 가능
1-3. 박싱(Boxing)과 언박싱(UnBoxing)
- 박싱(Boxing)과 언박싱(UnBoxing)
- 박싱(Boxing) : 기본타입을 래퍼클래스의 인스턴스로 인스턴스화 하는 것
- 언박싱(UnBoxin): 래퍼클래스 타입의 인스턴스를 기본 타입으로 변경하는 것
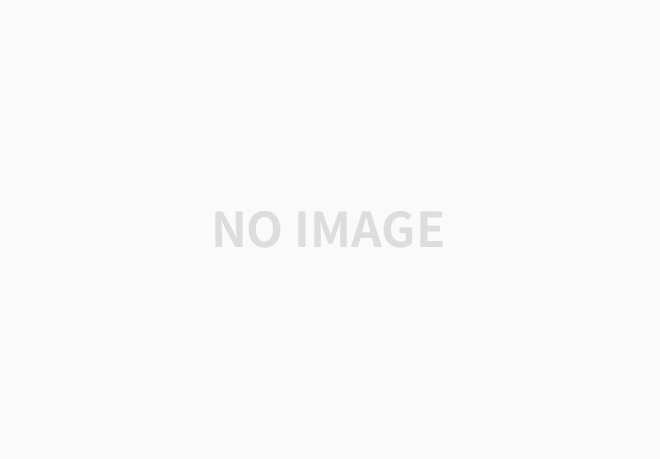
- 오토 박싱(AutoBoxing)과 오토 언박싱(AutoUnBoxing)
- JDK 1.5부터는 박싱과 언박싱이 필요한 상황에서 자바 컴파일러가 이를 자동으로 처리
- 자동화된 박싱과 언박싱을 오토 박싱, 오토 언박싱이라고 부른다.
package com.ohgiraffers.section04.wrapper;
public class Application1 {
public static void main(String[] args) {
/* 수업목표. Wrapper 클래스에 대해 이해할 수 있다. */
int intValue = 20;
/* 설명. 기본자료형을 Wrapper 클래스 자료형으로 변환할 수 있다.(박싱, boxing) */
Integer boxingInt = (Integer)20; // boxing (용어가 중요!) / up-casting 아니다!!!
Integer boxingInt2 = Integer.valueOf(intValue);
/* 설명. Wrapper 클래스 자료형을 기본자료형으로 변환할 수 있다.(언박싱, unboxing) */
int unboxingValue = boxingInt.intValue(); // 있구나 정도로만 알자. 자주 쓰므로 다른 방식이 있음.
/* 설명. 기본자료형과 Wrapper 클래스는 자동으로 박싱 및 언박싱이 일어난다. */ // 용어 알아두자!
Integer autoBoxingInt = intValue; // auto-boxing
int autoUnboxingInt = autoBoxingInt; // auto-unboxing
anythingMethod(10);
/* 설명. Wrapper 클래스 주소값 비교 */
Integer integerTest = 30;
Integer integerTest2 = 30;
System.out.println("== 비교: " + (integerTest == integerTest2));
System.out.println("equals() 비교: " + integerTest.equals(integerTest2));
System.out.println("integerTest 주소: " + System.identityHashCode(integerTest));
System.out.println("integerTest2 주소: " + System.identityHashCode(integerTest2));
System.out.println(integerTest.hashCode());
System.out.println(integerTest2.hashCode());
}
/* 설명. 매개변수가 Object인 메소드(어떤 자료형의 전달인자이든 받아낼 수 있는 메소드) */
public static void anythingMethod(Object obj) {
/* 설명. 10 -> Integer(오토박싱) -> Object(다형성) */
/* 설명. 출력 -> Object의 toString()에서(정적 바인딩) Integer의 toString()이(동적 바인딩)이 실행됨 */
System.out.println("obj: " + obj.toString()); // Object obj = new Integer(10); 이건가???????????????????
}
}
// 실행 결과
obj: 10
== 비교: true
equals() 비교: true
integerTest 주소: 1663383713
integerTest2 주소: 1663383713
30
30
package com.ohgiraffers.section04.wrapper;
public class Application2 {
public static void main(String[] args) {
/* 수업목표. 문자열을 다양한 기본 자료형으로 바꿀 수 있다. */
byte b = Byte.valueOf("1"); // "" + b -> 문자열로 바뀐다.
short s = Short.valueOf("2");
int i = Integer.valueOf("4");
long l = Long.valueOf("8");
float f = Float.valueOf("4.0");
double d = Double.valueOf("8.0");
boolean isTrue = Boolean.valueOf("true");
char c = "abc".charAt(0); // Character가 제공하지 않아 String의 charAt()을 사용
}
}
package com.ohgiraffers.section04.wrapper;
public class Application3 {
public static void main(String[] args) {
String b = Byte.valueOf((byte)1).toString(); // 1이 int로 byte 써줘야한다.
String s = Short.valueOf((short)2).toString();
String i = Integer.valueOf(4).toString();
String l = Long.valueOf(8L).toString();
String f = Float.valueOf(4.0f).toString();
String d = Double.valueOf(8.0).toString();
String isTrue = Boolean.valueOf(true).toString();
String ch = Character.valueOf('a').toString();
/* 설명. 기본자료형을 문자열로 바꿀 때는 간단히 ""을 더해주자 */
String floatString = 4.0f + "";
}
}
'한화시스템 > 백엔드' 카테고리의 다른 글
[BE] JAVA_예외처리 (0) | 2024.07.20 |
---|---|
[BE] JAVA_API_Time 패키지 (0) | 2024.07.20 |
[BE] JAVA_API_StringBuilder & StringBuffer (1) | 2024.07.19 |
[BE] JAVA_API_String (1) | 2024.07.19 |
[BE] JAVA_API_Object (0) | 2024.07.19 |